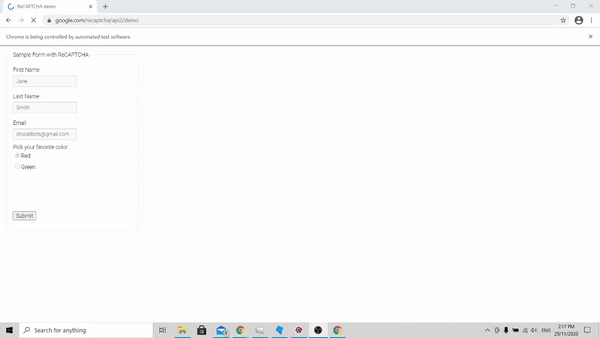
For those who learn better with video tutorial you can refer to my youtube link here: https://www.youtube.com/watch?v=Fdu81T9GgMA
Why I hate Recaptcha
Recaptcha is a huge roadblock for any automation engineers. It stops our bot from web scraping and blocks our automation software from booking our favorite concert tickets. This is done by asking our bot to solve “complicated” puzzles that only humans can apprehend. While the bot can choose to solve either the image or audio recognition challenge, both are equally challenging. Another way to solve this is to use Recaptcha Solver API from companies such as 2Captcha, where they employ humans to solve the ReCaptcha for you. Their service costs 0.0025USD per challenge.
Using AI to solve Recaptcha
With the advancement in AI and Machine learning technologies, we have amazing technologies such as Google Assistant, Telsa self-driving cars, and Deep Mind Alpha Go — the AI who defeated the world champion in 2016. So the question is, can we use these technologies to solve Recaptcha automatically for us? The short answer is YES, but it requires you to have some understanding in the field of AI and machine learning technologies.
Understanding AI Technologies
As I previously mentioned, there are two ways to solve the Recaptcha challenge (1) Image Recognition and (2) Audio Recognition.
(1) Image Recognition Challenge — This challenge requires the user to identify objects in a 3 x 3 frame. To solve this, it seems like a simple task for AI as we have many image classification algorithms such as GoogLeNet, ResNet, and MobileNet that can be trained to classify thousands of objects. So it shouldn’t be a problem to “select all images with a bicycle” right? Yes and no.
Yes, because it is simple for the algorithm to classify objects within one single frame, as shown in the figure below.

No, because the algorithm is not robust enough to identify the object when it is divided into many frames, as shown in the figure below.

(2) Audio Recognition Challenge — This challenge requires the user to listen to a sound clip and decipher the “human speech” from it. Using AI to detect human speech is a very mature technology, in a matter of fact, it has been integrated into Siri, Google Assistant, and Bixby to understand user commands. So can we use this method to solve Recaptcha? Yes!
This is the more preferred method as audio recognition is much simpler than image recognition, which yields lower computational resources and time.
Algorithm to bypass Recaptcha
For this tutorial, we will be bypassing a Recaptcha website provided by Google: https://www.google.com/recaptcha/api2/demo
Import the following libraries
#system libraries
import os
import random
import time#selenium libraries
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.support.ui import Select
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
from selenium.common.exceptions import TimeoutException
from selenium.common.exceptions import UnexpectedAlertPresentException
from selenium.webdriver.chrome.options import Options#recaptcha libraries
import speech_recognition as sr
import ffmpy
import requests
import urllib
import pydub
Step 1: Go to the Recaptcha website

def delay ():
time.sleep(random.randint(2,3))try:
#create chrome driver
driver = webdriver.Chrome(os.getcwd()+"\\webdriver\\chromedriver.exe")
delay()
#go to website
driver.get("https://www.google.com/recaptcha/api2/demo")
except:
print("[-] Please update the chromedriver.exe")
Step 2: Find the Recaptcha frame and click on the audio challenge

#switch to recaptcha frame
frames=driver.find_elements_by_tag_name("iframe")
driver.switch_to.frame(frames[0]);
delay()#click on checkbox to activate recaptcha
driver.find_element_by_class_name("recaptcha-checkbox-border").click()#switch to recaptcha audio control frame
driver.switch_to.default_content()
frames=driver.find_element_by_xpath("/html/body/div[2]/div[4]").find_elements_by_tag_name("iframe")
driver.switch_to.frame(frames[0])
delay()#click on audio challenge
driver.find_element_by_id("recaptcha-audio-button").click()#switch to recaptcha audio challenge frame
driver.switch_to.default_content()
frames= driver.find_elements_by_tag_name("iframe")
driver.switch_to.frame(frames[-1])
delay()#click on the play button
driver.find_element_by_xpath("/html/body/div/div/div[3]/div/button").click()
Step 3: Download audio challenge MP3 file
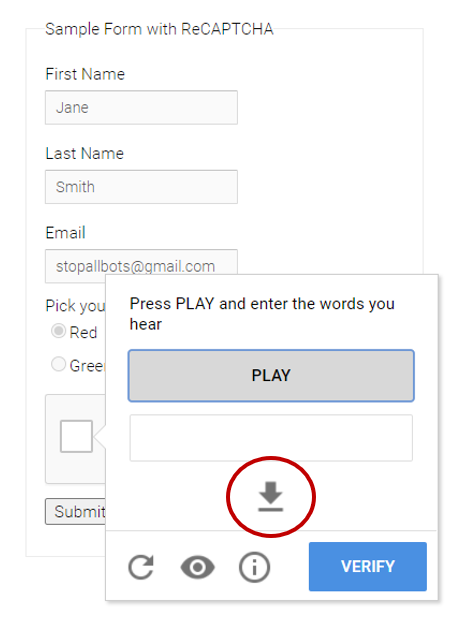
#get the mp3 audio file
src = driver.find_element_by_id("audio-source").get_attribute("src")
print("[INFO] Audio src: %s"%src)
#download the mp3 audio file from the source
urllib.request.urlretrieve(src, os.getcwd()+"\\sample.mp3")
Step 4: Convert from MP3 to WAV format
sound = pydub.AudioSegment.from_mp3(os.getcwd()+"\\sample.mp3")
sound.export(os.getcwd()+"\\sample.wav", format="wav")
sample_audio = sr.AudioFile(os.getcwd()+"\\sample.wav")
Step 5: Use Google speech to text API to decipher audio challenge
#translate audio to text with google voice recognition
key=r.recognize_google(audio)
print("[INFO] Recaptcha Passcode: %s"%key)
Step 6: Fill in the Recaptcha passcode and press verify

#key in results and submit
driver.find_element_by_id("audio-response").send_keys(key.lower())
driver.find_element_by_id("audio-response").send_keys(Keys.ENTER)
driver.switch_to.default_content()
delay()
driver.find_element_by_id("recaptcha-demo-submit").click()
delay()
Congratulation you have successfully bypass Google’s Recaptcha!
If you are unable to run the above-mentioned code you can follow my youtube tutorial for a more in-depth guide.
Github link: https://github.com/ohyicong/recaptcha_v2_solver
Other interesting articles
Disclaimer
I am sharing this knowledge to raise awareness of this vulnerability and demonstrate how easy this can be exploited. You should not use this tool on unauthorised websites. Cheers!